R Get Stock Price
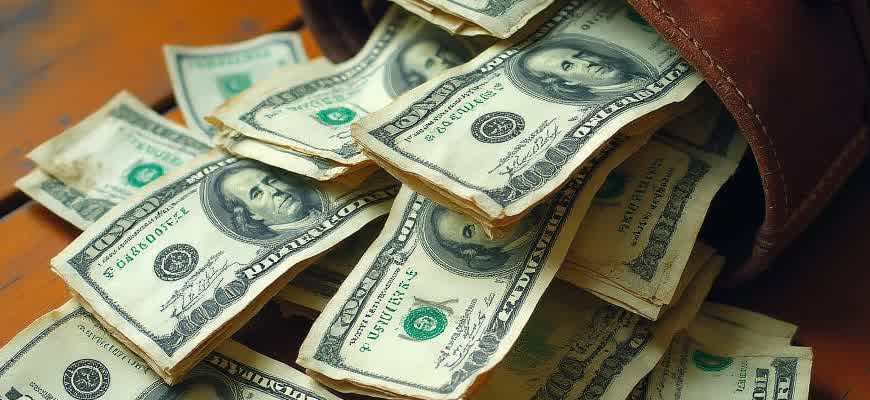
One of the most common tasks when analyzing cryptocurrency markets is retrieving real-time stock prices. The R programming language provides a variety of packages and methods for easily accessing financial data, including cryptocurrency prices. To get started, it's essential to understand the core tools that R offers to make such data retrieval possible and efficient.
To fetch the current price of a cryptocurrency using R, one can utilize the quantmod or tidyquant packages, which interface with financial data APIs. These packages allow users to pull data directly from sources like Yahoo Finance, Alpha Vantage, or other cryptocurrency-specific platforms.
- quantmod: Best known for fetching and modeling financial data.
- tidyquant: Provides a tidyverse approach to financial data, great for integration with other tidyverse packages.
- cryptor: Specially designed for working with cryptocurrency prices.
Important: Always check for API rate limits and ensure that you're using a key (if necessary) to avoid interruptions in data retrieval.
Here is an example of how to pull cryptocurrency data using the cryptor package in R:
library(cryptor) crypto_data <- crypto_price("bitcoin", "usd") print(crypto_data)
This simple script fetches the current price of Bitcoin in USD. Once you run it, you'll be able to analyze the most recent pricing information and make informed decisions for your investment strategy.
How to Retrieve Real-Time Cryptocurrency Data with R
For those looking to analyze cryptocurrency market trends, R provides various tools to gather real-time data. This can be done through several packages that interact with APIs to fetch live data, such as the current price, market cap, volume, and more. With this information, you can perform detailed analyses or backtest trading strategies to better understand market movements.
To get started, you need to have a basic understanding of how to connect to an API, process the data, and visualize the results. In this guide, we’ll explore the process of fetching live cryptocurrency data from sources like CoinGecko or CryptoCompare using R programming language.
Steps to Fetch Real-Time Crypto Data
- Install Required Packages: Start by installing packages such as httr or jsonlite for making API calls and parsing JSON responses.
- Set Up the API Endpoint: Choose a data provider that offers free access to cryptocurrency data, like CoinGecko's public API.
- Extract Data: Use the API to pull live data. Typically, the API will return information in JSON format, which you will then parse and store for analysis.
- Visualize the Data: Once data is retrieved, you can use R’s ggplot2 or plotly to visualize price movements over time.
Example of Using CoinGecko API
Here’s an example of how to retrieve the current price of Bitcoin using the CoinGecko API in R:
library(httr) library(jsonlite) # Define the API endpoint url <- "https://api.coingecko.com/api/v3/simple/price?ids=bitcoin&vs_currencies=usd" # Fetch the data response <- GET(url) data <- fromJSON(content(response, "text")) # Extract the price of Bitcoin bitcoin_price <- data$bitcoin$usd # Print the result print(paste("Current Bitcoin price:", bitcoin_price))
Important Notes
Ensure you follow the API's usage limits to avoid being blocked. Some APIs have rate limits that restrict how many requests you can make in a given time period.
API Response Example
Field | Value |
---|---|
id | bitcoin |
currency | USD |
price | 43210.56 |
Integrating APIs for Real-Time Cryptocurrency Price Monitoring
In the rapidly evolving world of cryptocurrency, obtaining accurate and real-time market data is crucial for investors, traders, and analysts. Integrating Application Programming Interfaces (APIs) for real-time price updates provides a seamless solution to track price movements of various cryptocurrencies. These APIs offer direct access to live market data, making it easier for platforms or individuals to monitor price fluctuations continuously without relying on outdated or delayed sources.
To efficiently integrate these APIs, it's important to choose services that provide accurate, up-to-the-minute information. The most commonly used APIs in the crypto space include CoinGecko, CoinMarketCap, and Binance. These platforms offer extensive data, from current prices and trading volumes to historical data and market cap details, giving users the ability to make informed decisions on their investments.
Steps for API Integration
- Choose an API provider that offers the necessary data for your needs (e.g., CoinGecko, CoinMarketCap, Binance).
- Register for an API key on the platform’s website.
- Implement the API using standard protocols like RESTful services.
- Ensure that your system handles updates in real-time to reflect the most accurate prices.
- Verify data accuracy by testing with multiple API responses.
Important Considerations
Real-time data can be volatile, so it's essential to have a mechanism in place that handles errors or discrepancies when data updates are delayed or unavailable.
Additionally, some APIs offer different rate limits based on subscription plans. Higher subscription tiers often provide more frequent updates and access to advanced features. It's important to factor in these limitations when designing a system for continuous data fetching to avoid disruptions.
Comparison of Common APIs for Cryptocurrency Prices
API Provider | Supported Cryptos | Update Frequency | Free Tier |
---|---|---|---|
CoinGecko | 2000+ | Real-time | Yes |
CoinMarketCap | 2000+ | Real-time | Yes (limited) |
Binance | 500+ | Real-time | Yes (limited) |
The choice of API can significantly impact the accuracy and speed of the data, so consider your project's scale when selecting a provider.
Challenges in Managing Cryptocurrency Data with R
Handling cryptocurrency market data within R presents unique challenges compared to traditional stock market data. Unlike stocks, which are primarily traded on centralized exchanges, cryptocurrencies are traded on numerous decentralized platforms, leading to fragmented data sources. This fragmentation creates difficulties in obtaining consistent and reliable data for analysis. Additionally, the high volatility and 24/7 nature of cryptocurrency markets add layers of complexity when it comes to data accuracy and processing.
Another significant challenge is the issue of data formatting and synchronization. Cryptocurrency data comes in various formats, often without a standardized API, making it challenging to process and analyze efficiently. In R, common tasks like aggregating data from different exchanges or aligning timestamps can become time-consuming, especially if data from different platforms needs to be merged or cleaned.
Data Quality and Integrity Issues
Ensuring data accuracy is crucial when dealing with cryptocurrency. Inaccuracies can arise due to data exchange discrepancies, API malfunctions, or inconsistent updates. This is especially problematic when performing technical analysis or building predictive models. Below are key points to consider:
- Data Source Consistency: Different exchanges may report slightly different prices for the same cryptocurrency due to variations in trading volume and liquidity.
- Price Spikes and Outliers: Cryptocurrencies often experience sudden price fluctuations that can distort analysis if not handled properly.
- Missing Data: Gaps in data are common, especially when exchanges experience downtime or API issues.
Handling Time Series Data
Cryptocurrency data often comes with irregular timestamps and differing time intervals across data sources. Time series analysis in R can be complicated when data points are not evenly spaced, requiring careful preprocessing before any meaningful analysis can be performed. For example, the following challenges may arise:
- Time Synchronization: Aligning data across different time zones or exchanges can be difficult, especially if timestamps are not uniform.
- Frequency Resampling: Resampling data to a uniform time frequency (e.g., hourly or daily) may lead to loss of valuable information.
- High-Frequency Data: Managing minute- or second-level cryptocurrency data can result in large datasets, requiring efficient storage and processing techniques.
Accurate and timely data is essential for any predictive modeling in the cryptocurrency space. Errors in the dataset can lead to misleading insights, which may result in poor trading decisions.
Summary of Common Challenges
Challenge | Impact |
---|---|
Data Source Discrepancies | Inconsistent prices across exchanges |
Data Integrity Issues | Inaccurate analysis or misleading trends |
Missing or Incomplete Data | Invalid conclusions or model errors |
Time Series Alignment | Inconsistent data analysis and forecasting |
Analyzing and Visualizing Cryptocurrency Trends with R: A Comprehensive Guide
Cryptocurrency markets are volatile, making it essential for traders and analysts to track price trends effectively. One way to do this is by utilizing R, a powerful tool for data analysis and visualization. By leveraging various packages, R allows users to analyze and visualize cryptocurrency trends, providing insights into market behavior. With a well-structured approach, you can import, clean, and transform crypto price data before generating meaningful visualizations.
In this guide, we will explore how to visualize cryptocurrency price trends using R. From acquiring historical data to creating dynamic plots, we will break down each step to ensure you can effectively track and interpret market movements. Below is an overview of the process, focusing on key tools and functions.
Step-by-Step Process for Visualizing Cryptocurrency Prices
- Acquiring Data: Start by gathering historical cryptocurrency price data. You can use APIs like CryptoCompare or CoinGecko to fetch live data. Another option is using the quantmod package to pull data directly into R.
- Data Cleaning: After fetching the data, clean it by removing missing values and ensuring the data is in a proper format for analysis.
- Visualization: Utilize the ggplot2 package for creating plots. You can generate line charts, candlestick plots, or time series graphs to illustrate price movements over time.
For example, a simple line plot can be created to show the daily closing price of Bitcoin. The code snippet below demonstrates how to do this:
library(ggplot2) # Assuming 'crypto_data' is a cleaned dataset with 'date' and 'close' columns ggplot(crypto_data, aes(x = date, y = close)) + geom_line() + labs(title = "Bitcoin Price Trend", x = "Date", y = "Closing Price (USD)")
"Visualization helps to identify key patterns, such as bull or bear markets, making it an indispensable tool for cryptocurrency traders."
Key Points to Keep in Mind
- Ensure that the data you import is up-to-date and accurate for reliable analysis.
- Consider the use of moving averages to smooth out price data for better trend identification.
- Integrate other indicators like Relative Strength Index (RSI) or Bollinger Bands for deeper insights into market conditions.
Example: Cryptocurrency Price Data Table
Date | Open Price | Close Price | High | Low |
---|---|---|---|---|
2025-04-01 | $28,000 | $29,500 | $30,000 | $27,800 |
2025-04-02 | $29,500 | $30,000 | $31,000 | $28,000 |
2025-04-03 | $30,000 | $29,800 | $31,500 | $28,700 |
Enhancing Data Retrieval Efficiency for Cryptocurrency Prices in R
In the world of cryptocurrency, having real-time access to accurate price data is crucial for traders and analysts. When working with R to fetch stock or crypto prices, the efficiency of data retrieval can greatly impact the overall workflow and performance. Optimizing the process can save time and improve the accuracy of decision-making, especially when dealing with large datasets and high-frequency updates. In this context, minimizing latency and maximizing speed are essential objectives.
One of the primary methods to enhance the data fetching process is by using APIs that are optimized for high-speed access. Additionally, leveraging efficient libraries and minimizing unnecessary calls can significantly boost performance. Some techniques, such as caching frequently requested data, can also play a key role in reducing redundant operations.
Key Strategies for Optimizing Data Retrieval in R
- Utilize Asynchronous Requests: Asynchronous operations allow you to fetch data without blocking the execution of other tasks, enabling simultaneous queries for multiple assets.
- Implement Caching: By caching results from frequently accessed data points, you can avoid redundant API calls, thereby improving speed.
- Use Efficient Libraries: Libraries like 'quantmod' and 'tidyquant' are specifically designed for financial data and optimized for faster data retrieval.
- Limit API Call Frequency: Setting appropriate time intervals for API calls reduces unnecessary load on the server and improves response time.
Tools for Efficient Crypto Price Fetching
- Cryptowatch API: Provides high-quality real-time price data with low-latency endpoints.
- Binance API: A popular choice for fetching cryptocurrency price data with excellent scalability and speed.
- CoinGecko API: Known for offering fast access to historical and real-time cryptocurrency prices with high reliability.
Important Tip: Always monitor the rate limits and request quotas of the API you are using to prevent throttling and ensure consistent performance.
Example of Efficient Data Fetching
API | Latency | Rate Limit | Data Coverage |
---|---|---|---|
Cryptowatch | Low | 200 requests/min | Real-time, 50+ crypto pairs |
Binance | Moderate | 1200 requests/min | Spot & futures data |
CoinGecko | Low | 50 requests/sec | Historical & real-time |
Utilizing R for Cryptocurrency Price Analysis: Insights from Historical Data
In the world of cryptocurrency, historical data analysis is crucial for making informed trading decisions. Leveraging R to analyze past price movements can help investors and analysts identify patterns, trends, and potential future outcomes. By collecting and processing large datasets from various cryptocurrency exchanges, R provides a powerful environment for conducting time series analysis and modeling volatility, which is common in digital asset markets.
R offers a range of tools and libraries, such as `quantmod` and `tidyquant`, that make it easy to pull cryptocurrency data from sources like CoinGecko or Binance. Once data is retrieved, users can visualize historical price trends, calculate returns, and assess the impact of external factors such as market events or regulatory changes.
Steps for Analyzing Historical Cryptocurrency Data in R
- Data Retrieval: Use APIs to fetch historical cryptocurrency data, including prices, volume, and market cap.
- Data Cleaning: Prepare the data by handling missing values, adjusting for splits, and formatting it for analysis.
- Time Series Analysis: Apply time series techniques to study trends, seasonality, and volatility.
- Visualization: Generate graphs and charts to display price movements, moving averages, and other statistical indicators.
R allows for complex statistical analyses and visualizations, making it ideal for modeling the often volatile and unpredictable behavior of cryptocurrency markets.
Key Metrics to Consider in Crypto Analysis
- Price Movements: The price history of a cryptocurrency gives insights into its volatility and long-term trends.
- Volume Data: Trading volume can help gauge market sentiment and potential price movements.
- Market Capitalization: This metric provides an overall view of a cryptocurrency’s market dominance and size.
- Volatility: Crypto assets are known for their high volatility, which can be analyzed using standard deviation or the VIX index.
Metric | Description |
---|---|
Price | Current trading value of the cryptocurrency. |
Volume | The total amount of the cryptocurrency traded over a specified period. |
Market Cap | The total value of all circulating coins/tokens in the market. |
Volatility | Measures the degree of price fluctuations over time. |
Customizing Cryptocurrency Price Queries for Different Markets
When querying cryptocurrency prices, it's important to adjust your queries to target specific exchanges, as each exchange may have different data structures, API access protocols, and rate limits. Understanding how to customize queries for these exchanges ensures that you can access accurate and relevant data tailored to your needs. Below, we discuss various ways to optimize price queries, particularly for specialized markets and crypto assets.
For instance, major exchanges like Binance, Coinbase, and Kraken have different API endpoints and parameters that must be incorporated into the query structure. Additionally, smaller or regional exchanges may offer unique cryptocurrencies or specialized pairs that are not listed on larger platforms. Customizing these queries allows you to gather data specific to the asset's performance on those exchanges, leading to more accurate financial analysis and trading decisions.
Key Steps for Customizing Queries
- API Access and Authentication: Ensure that you have valid API keys to access exchange-specific data.
- Endpoint Configuration: Select the correct endpoint that corresponds to the exchange and cryptocurrency pair you want to monitor.
- Rate Limits: Pay attention to the rate limits imposed by the exchange's API to avoid data fetching issues.
- Data Filters: Filter the data based on specific parameters such as trading volume, time frames, or price range.
By customizing your queries, you can receive tailored data that improves your trading strategy and decision-making process. Make sure to stay updated on changes in API protocols and exchange features to maintain seamless data retrieval.
Example of Customized Query Structure
Below is an example of how to set up a customized query to fetch the price of Bitcoin (BTC) from the Binance exchange:
- Obtain the API key from Binance's developer portal.
- Construct the API request URL:
https://api.binance.com/api/v3/ticker/price?symbol=BTCUSDT
. - Apply any necessary filters, such as adjusting the time window or specifying the exact price format (USD, EUR, etc.).
- Handle the returned data (e.g., timestamp, price) in your application or script.
By modifying the query URL, you can adjust the cryptocurrency pair or even query a specific exchange’s trading fees or order book data.
Example Data Format
Field | Value |
---|---|
Symbol | BTCUSDT |
Price | 42312.45 |
Timestamp | 2025-04-20T15:30:00Z |
Automating Cryptocurrency Price Retrieval and Notifications in R
With the growing interest in cryptocurrencies, it is crucial to stay updated with their price movements. Automating the retrieval of crypto price data using R can save time and enhance decision-making. This can be accomplished using APIs, libraries, and custom scripts that regularly fetch real-time prices. Additionally, integrating notification systems can alert users when specific price thresholds are met, adding a layer of convenience and efficiency to cryptocurrency trading or monitoring.
One effective approach involves using R packages such as `httr` for API calls and `jsonlite` for parsing JSON responses. With these tools, users can connect to popular cryptocurrency data sources like CoinGecko or CryptoCompare. Furthermore, implementing email or SMS notifications ensures that users are alerted promptly about price changes, enabling timely actions in a volatile market.
Steps for Automating Cryptocurrency Price Monitoring
- Set up API keys from cryptocurrency data providers.
- Use R to fetch live price data in real-time.
- Parse the returned JSON data for specific information (e.g., price, volume, market cap).
- Implement price comparison logic to trigger notifications.
- Set up email or SMS notifications based on defined price thresholds.
Below is an example of how to implement a simple automated script that fetches the current price of Bitcoin using the CoinGecko API:
library(httr) library(jsonlite) response <- GET("https://api.coingecko.com/api/v3/simple/price?ids=bitcoin&vs_currencies=usd") data <- fromJSON(content(response, "text")) bitcoin_price <- data$bitcoin$usd print(paste("Current Bitcoin price is:", bitcoin_price))
Tip: To ensure reliability, schedule the script to run at fixed intervals using task schedulers such as cron jobs (Linux) or Task Scheduler (Windows).
Sample Table: Notification Criteria for Cryptocurrency Prices
Cryptocurrency | Price Threshold (USD) | Notification Type |
---|---|---|
Bitcoin | 60000 | |
Ethereum | 4000 | SMS |
Ripple | 1.5 |
Reminder: Regularly check API rate limits to avoid service disruptions.