Boost Tokenizer String_view
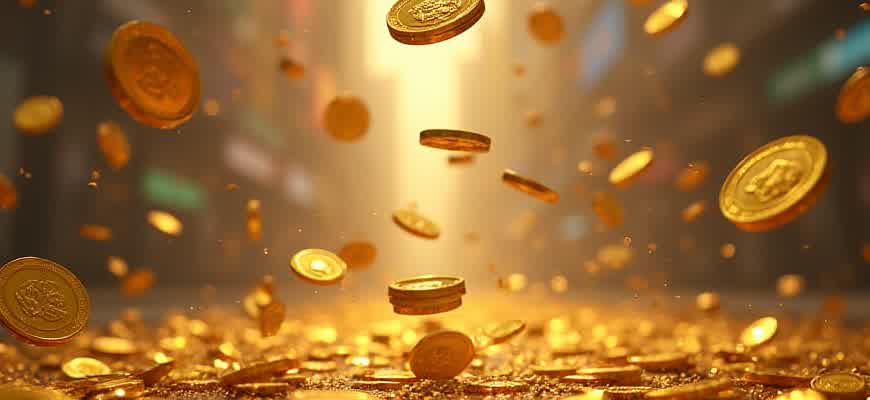
The Boost Tokenizer library provides a versatile solution for text processing, especially in scenarios that require efficient token extraction. By integrating the String_View feature, which allows non-copying, low-overhead manipulation of strings, developers can achieve higher performance when parsing large datasets or strings in cryptographic applications.
String_View ensures that tokens are viewed directly in memory without the need for creating additional substrings, which can significantly reduce both time and space complexity in applications. This optimization is particularly useful in cryptocurrency-related tools where processing speed is critical.
Key Benefit: By utilizing String_View, unnecessary memory allocations are avoided, improving the overall performance of the tokenizer.
Below are some of the advantages of combining Boost Tokenizer with String_View:
- Memory Efficiency: No copying of string data.
- Faster Token Parsing: Optimized for large text processing.
- Seamless Integration: Works smoothly with existing Boost libraries.
To highlight the improvements in performance, consider the following example where String_View is utilized:
Feature | Traditional Tokenizer | Tokenizer with String_View |
---|---|---|
Memory Usage | Higher (due to substring allocations) | Lower (direct string access) |
Performance | Slower on large inputs | Faster on large inputs |
Flexibility | Limited | Highly flexible for different data structures |
Optimizing Memory Usage in String Handling with String_view
Efficient memory management is crucial in the development of cryptocurrency applications, especially when dealing with large sets of data, such as transaction logs or blockchain records. One technique to optimize memory usage in string handling is the use of `std::string_view`, a feature available in C++ that allows non-owning references to string data without requiring costly copies. This is especially beneficial in performance-sensitive applications like blockchain explorers or decentralized exchange backends, where the overhead of memory allocation can significantly impact efficiency.
Using `std::string_view`, developers can manipulate portions of strings without the need to duplicate the data, leading to reduced memory usage and faster execution times. By referencing data instead of copying it, applications can avoid the common pitfalls of memory bloat and ensure smoother performance under high load. This is particularly important when parsing large JSON objects or processing transaction strings on a blockchain network.
Key Benefits of String_view in Cryptocurrency Development
- Memory Efficiency: Avoids unnecessary allocations by referencing string data directly.
- Improved Performance: Reduces the overhead associated with copying large data sets.
- Non-owning Semantics: Strings are accessed without ownership, minimizing resource management complexity.
Example Use Case in Blockchain Transaction Parsing
When parsing blockchain transaction data, which often includes lengthy strings such as addresses, hashes, and signatures, using `std::string_view` can provide substantial memory savings. Consider a situation where only parts of a string (like a transaction hash) are needed. Instead of copying the entire string, a `std::string_view` can be used to reference the relevant portion, keeping memory usage minimal.
Operation | With std::string | With std::string_view |
---|---|---|
Memory Allocation | Full copy of data | No copy, just a reference |
Performance | Higher overhead | Faster processing |
Data Ownership | Owns the data | No ownership |
Important: The use of `std::string_view` is best suited for situations where string data will not be modified and must not outlive the original data source. Ensure that the lifetime of the underlying string is managed carefully to avoid dangling references.
Leveraging Boost Tokenizer with String_view for Real-Time Cryptocurrency Applications
In the realm of cryptocurrency, real-time processing of market data and transaction logs is crucial for maintaining accuracy and efficiency. The integration of Boost Tokenizer with C++’s String_view allows developers to handle large datasets, such as blockchain transactions or market updates, with minimal overhead. By working with String_view, which does not require data copying, and leveraging Boost Tokenizer for token parsing, applications can achieve faster processing times while reducing memory footprint. This becomes especially valuable when dealing with the high-frequency data streams commonly found in cryptocurrency systems.
Real-time systems need high performance and low latency, both of which are key benefits when using Boost Tokenizer in conjunction with String_view. This combination allows applications to parse incoming data quickly without unnecessary memory allocations, a crucial factor when processing large volumes of messages or blocks of transactions. For instance, parsing raw transaction data from a blockchain or handling incoming trading signals in cryptocurrency markets can be optimized using this integration.
Key Advantages
- Memory Efficiency: String_view avoids copying data, improving memory management during real-time parsing operations.
- High Performance: Tokenization with Boost Tokenizer allows fast and efficient parsing of data streams, crucial for real-time applications.
- Low Latency: The integration ensures that tokenization does not introduce significant delays, maintaining system responsiveness.
Implementation Considerations
- Data Stream Parsing: A common use case is parsing cryptocurrency transactions from a raw data stream. Boost Tokenizer can split the stream into meaningful tokens (e.g., transaction ID, timestamp, amount).
- Efficiency in Handling JSON Responses: Many cryptocurrency APIs return data in JSON format. String_view allows developers to efficiently parse the data without copying the raw input.
- Integration with Blockchain Networks: Real-time applications such as blockchain explorers can use this technique to efficiently parse and display transaction data in real time.
Example Use Case: Parsing Blockchain Transactions
Token | Value |
---|---|
Transaction ID | abc123xyz456 |
Timestamp | 1617283500 |
Amount | 0.25 BTC |
"Efficient real-time transaction parsing is critical in cryptocurrency networks, where delays can lead to substantial losses. Boost Tokenizer with String_view provides a robust solution for high-performance data parsing."
Customizing Delimiters in Boost Tokenizer for Efficient Parsing
Boost Tokenizer provides a versatile mechanism for breaking down strings into tokens, making it an essential tool for text processing in various applications, including cryptocurrency parsing. In many cases, the default delimiter set may not fit the specific needs of a use case, especially when handling complex data formats like transaction logs, cryptocurrency addresses, or blockchain data. Customizing delimiters allows fine-grained control over the tokenization process, ensuring that each element is correctly parsed without unnecessary overhead.
By configuring custom delimiters, developers can tailor the tokenizer to handle a variety of characters or sequences, enabling more accurate and context-specific tokenization. For example, when processing data that includes specific symbols or delimiters like commas, colons, or even spaces, adjusting the delimiter rules can make the tokenizer more efficient and aligned with the application's requirements.
Steps to Customize Delimiters
To set custom delimiters in Boost Tokenizer, you'll need to adjust the delimiter function used by the tokenizer object. Here’s an outline of how this can be done:
- Define Custom Delimiters: Create a set of characters or patterns that will act as your delimiters. This can include common symbols or specific character sequences.
- Update Tokenizer Configuration: Use the custom delimiters within the tokenizer setup. The tokenizer can accept either a character or regular expression to identify split points.
- Handle Edge Cases: Test edge cases where delimiters might overlap or where special characters are involved, ensuring that the tokenizer correctly handles these scenarios.
Important: If the delimiter set includes regular expressions, be sure to escape special characters properly to avoid unexpected behavior during parsing.
Example Table: Tokenization with Custom Delimiters
String | Custom Delimiters | Expected Tokens |
---|---|---|
"BTC:100, ETH:50" | ":" and "," | BTC, 100, ETH, 50 |
"Alice -> Bob: 10" | " " and "->" | Alice, Bob, 10 |
When properly configured, custom delimiters ensure that tokenization matches the intended data structure, such as transactions, block data, or cryptocurrency wallet addresses, thereby facilitating smooth data extraction and further processing.
Leveraging Boost Tokenizer in Multithreaded Cryptographic Applications
In modern multithreaded applications, especially in the context of blockchain and cryptocurrency systems, processing large volumes of data concurrently is crucial. Tokenization, the process of dividing a stream of text or data into meaningful segments, plays a critical role in parsing transactions, addresses, and other structured data within blockchain networks. Boost Tokenizer provides a highly efficient and scalable way to tokenize data streams in parallel environments, helping developers optimize the performance of cryptocurrency applications. Its integration with string views offers minimal memory overhead, making it suitable for high-performance systems where low latency is essential.
By utilizing Boost Tokenizer with multithreading, developers can significantly speed up the processing of incoming transaction data, ensuring that blockchain nodes or cryptocurrency exchanges handle high transaction throughput without bottlenecks. With the advent of parallel computing, leveraging concurrent tokenization has become an essential practice for maintaining real-time performance and scalability in decentralized financial systems. Boost Tokenizer’s thread-safety and fine-tuned performance under multithreaded conditions make it an ideal tool for this purpose.
Key Benefits of Boost Tokenizer in Multithreaded Environments
- Reduced Latency: Boost Tokenizer minimizes tokenization overhead by operating directly on string views, ensuring faster parsing of transaction data.
- Memory Efficiency: String views allow tokenization without the need for copying data, making the approach memory-efficient.
- Thread-Safety: With built-in support for concurrent execution, Boost Tokenizer ensures that multiple threads can safely process data without causing race conditions.
Implementation Considerations
When integrating Boost Tokenizer into multithreaded cryptocurrency systems, it is important to properly manage thread synchronization to prevent data inconsistencies. Using a thread pool can significantly improve performance, as it allows the system to manage a limited number of threads efficiently. Developers should also consider thread-local storage to minimize contention when working with large data sets.
Optimizing the interaction between Boost Tokenizer and multithreading frameworks such as Intel TBB or OpenMP can yield substantial improvements in throughput and response times in high-frequency trading platforms.
Comparison of Tokenization Methods in Multithreaded Cryptocurrency Applications
Method | Efficiency | Thread-Safety | Memory Overhead |
---|---|---|---|
Boost Tokenizer | High | Yes | Low |
Custom Tokenizer | Medium | Depends on Implementation | Medium |
Regular Expressions | Low | No | High |