Token C Programming
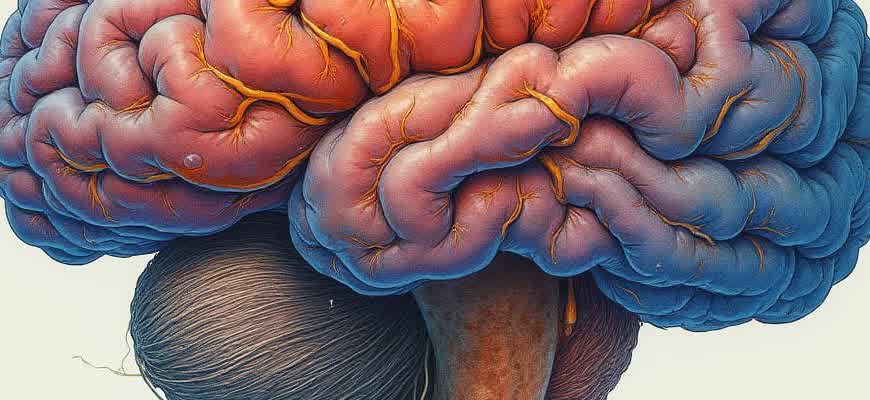
Token programming in C language plays a pivotal role in cryptocurrency development, especially in defining and managing digital assets. In the context of blockchain, tokens are the digital representation of assets or utilities that are built on top of a base blockchain protocol. The C programming language, being a low-level and performance-oriented language, provides the foundation for creating highly efficient token systems and smart contracts.
To understand how token programming functions in C, it's essential to comprehend the basic structure and components involved. Here's a quick breakdown of the core elements:
- Token Definition: Tokens are defined through specific data structures in C that outline the properties, behaviors, and values of the token.
- Transaction Handling: The logic for handling transactions, including creation, transfer, and validation, is built using C's control flow and data manipulation features.
- Security Measures: C allows direct memory management, which can be leveraged to implement robust cryptographic protocols for secure token transactions.
"Token programming in C demands a deep understanding of both low-level system programming and cryptographic principles, enabling the development of fast and secure blockchain solutions."
In addition to the token's structure, developers need to manage transaction flow and ensure the integrity of the system. A typical C token implementation might include:
- Transaction verification
- Account balances management
- Cryptographic signing and validation
Here is a simplified comparison of different aspects in token programming with C:
Feature | C Token Programming | Other Languages |
---|---|---|
Memory Management | Manual control | Automatic garbage collection |
Performance | Highly optimized | Less efficient |
Security | Customizable cryptography | Prebuilt security libraries |
How to Set Up Your Token C Development Environment
Creating a robust development environment for token creation on the C programming platform is crucial for building a reliable and efficient blockchain application. The setup involves various steps, from configuring the right tools to ensuring the proper dependencies are installed. This guide will help you walk through the process, ensuring you have everything ready to start developing your token application.
In order to start developing with Token C, you'll need to ensure that your machine is equipped with the necessary libraries, compilers, and other essential software. Here’s a step-by-step process for setting up a development environment for your cryptocurrency token project.
Step-by-Step Guide
- Install C Compiler
Make sure you have a C compiler installed on your machine. Common compilers include GCC for Linux and Clang for macOS. On Windows, MinGW or MSYS2 can be used to install a compatible C compiler.
- Set Up the Token Framework
Choose an appropriate framework or library that supports blockchain token development. For C programming, you might consider integrating the OpenSSL library for encryption and cryptographic functions.
- Download and Configure Dependencies
Use a package manager like apt for Linux or Homebrew for macOS to install all dependencies. Ensure libraries such as libcurl for network requests and JSON-C for JSON parsing are available in your environment.
Required Tools
- GCC Compiler – Essential for compiling C code and libraries.
- OpenSSL – Used for cryptographic operations and securing transactions.
- libcurl – Handles HTTP requests, often used for interacting with blockchain nodes.
- JSON-C – Useful for working with JSON data structures in your token operations.
Development Environment Setup Table
Tool | Platform | Installation Command |
---|---|---|
GCC | Linux / macOS | sudo apt install gcc |
OpenSSL | Linux / macOS | sudo apt install libssl-dev |
libcurl | Linux / macOS | sudo apt install libcurl4-openssl-dev |
JSON-C | Linux / macOS | sudo apt install libjson-c-dev |
Important: Ensure all dependencies are installed and configured before starting your project. Missing libraries could lead to errors and instability in your token development.
Key Differences Between Token C and Traditional C Programming
Token C programming has emerged as a specialized version of the traditional C language, designed to interact with blockchain platforms and smart contracts. The primary focus of Token C is enabling the creation, management, and interaction with digital tokens in decentralized environments. Traditional C, on the other hand, is a general-purpose language primarily used for system and application software development, with no direct interaction with blockchain technologies. These differences highlight how the two approaches serve different purposes and environments.
While both languages share common C syntax, Token C introduces specific features that enable secure and efficient token creation, contract execution, and blockchain interaction. Below are some of the main distinctions between Token C and traditional C programming, reflecting their respective use cases in blockchain and general computing.
Key Differences
- Focus on Blockchain Integration: Token C is tailored for smart contracts and token management on blockchain networks. Traditional C is optimized for building high-performance applications that run on centralized systems.
- Memory Management: Token C typically operates in a controlled, sandboxed environment provided by the blockchain platform, which abstracts away many low-level memory management tasks. Traditional C gives developers full control over memory allocation and deallocation, making it more complex but also more flexible.
- Execution Environment: Token C operates within a decentralized and transparent environment, ensuring that smart contracts are verifiable and immutable. Traditional C programs run on centralized systems, often without such transparency or security guarantees.
Blockchain-Specific Features in Token C
- Smart Contract Development: Token C allows developers to write and deploy smart contracts directly onto blockchain networks, enabling secure, automatic execution of terms defined by the contract.
- Cryptographic Functions: Token C integrates cryptographic operations like digital signatures and hash functions as part of its core functionality, making it easier to interact with tokens and secure transactions.
- Gas and Transaction Costs: Operations written in Token C often require "gas," a measure of computational effort to perform actions on the blockchain, which affects transaction costs. Traditional C does not involve such transaction fees.
Comparison Table
Feature | Token C | Traditional C |
---|---|---|
Purpose | Blockchain smart contracts, token management | General-purpose software development |
Memory Management | Automated, abstracted in blockchain environment | Manual, requires explicit memory handling |
Execution Environment | Decentralized, blockchain-based | Centralized, local machine or server |
Transaction Costs | Involves gas fees for execution | No transaction costs |
Important: Token C is specifically designed for the blockchain ecosystem, enabling developers to manage tokens, execute smart contracts, and interact with decentralized networks efficiently. Traditional C is better suited for local applications that do not require blockchain-based functionality.
How to Connect Token C with Blockchain Projects
Integrating Token C into blockchain applications requires understanding both the token standards and the technical requirements for seamless operation. Token C is a unique solution designed to simplify the implementation of cryptocurrency-based projects by offering an easy-to-use API and a secure environment for token creation. Blockchain developers can leverage Token C to issue their own tokens, enabling a variety of decentralized applications (dApps) to function without the complexity of creating a custom solution from scratch.
To successfully implement Token C into a blockchain project, developers must follow a few essential steps. This process includes configuring the smart contract on a blockchain, setting up token functionalities, and ensuring compliance with security protocols. Token C’s features allow projects to manage tokens for various use cases, such as governance, staking, and transfers, within an established blockchain ecosystem.
Steps to Integrate Token C
- Smart Contract Development: Create a smart contract that defines the token logic. Token C allows developers to customize token behaviors, such as transfers, minting, and burning, according to project needs.
- Blockchain Compatibility: Ensure the blockchain you are working with supports Token C standards. Popular blockchains like Ethereum, Binance Smart Chain, and Polygon are compatible.
- Security and Audits: Conduct thorough security audits on the smart contract to prevent vulnerabilities, ensuring that the token operates safely in the decentralized environment.
- Token Distribution: Define the initial supply, distribution model, and potential use cases for the token within your blockchain ecosystem.
Example of Token C Integration
Step | Action |
---|---|
Step 1 | Define Token Parameters (name, symbol, decimals, etc.) |
Step 2 | Deploy Token C smart contract on the desired blockchain network |
Step 3 | Integrate token functions with your dApp for transactions |
Note: Token C offers modularity, meaning it can be adapted for a wide range of use cases within the blockchain ecosystem, from DeFi protocols to NFT platforms.
Optimizing Performance in Token C-based Smart Contracts
Smart contracts written in C-based languages play a crucial role in the blockchain ecosystem, especially when it comes to token operations. However, optimizing the performance of these contracts is essential to ensure scalability, security, and efficiency. Given the increasing complexity of decentralized applications (dApps), developers must focus on specific areas to minimize resource consumption and enhance execution speed. Here, we discuss various techniques for achieving optimal performance in C-based token contracts, including memory management, gas efficiency, and computational optimization.
The performance of C-based token contracts can significantly impact transaction costs and user experience. By addressing common inefficiencies, developers can create contracts that run faster and use fewer resources while maintaining security. Below are key strategies for improving the performance of token contracts:
Key Optimization Strategies
- Efficient Memory Allocation: Proper management of memory can reduce the overhead of smart contract execution. Minimizing dynamic memory allocations and avoiding memory fragmentation are essential for enhancing contract speed.
- Gas Cost Reduction: Optimizing functions to lower gas consumption is critical for token contracts, as excessive gas fees can discourage users from interacting with the contract.
- Code Complexity Minimization: Simplifying contract logic can reduce execution times, leading to faster processing and lower operational costs.
Important Techniques for Performance Enhancement
- Pre-calculation of Constants: Pre-calculating values and storing them in variables avoids redundant computations during contract execution.
- Efficient Data Storage: Using compact data structures and minimizing state changes can prevent unnecessary on-chain storage, saving both gas and time.
- Optimizing Loops and Conditional Statements: Carefully optimizing loop structures and conditional checks can drastically reduce unnecessary iterations.
Note: Reducing the number of state updates and external calls within smart contracts can greatly improve transaction throughput and decrease operational costs.
Performance Benchmarking
Optimization Technique | Impact |
---|---|
Efficient Memory Management | Reduces memory overhead and improves execution speed. |
Gas Fee Optimization | Lowers transaction fees and improves user experience. |
Code Refactoring | Minimizes code complexity, resulting in faster execution. |
Security Considerations for Token C Code in Distributed Applications
When developing distributed applications with token-based systems, particularly those using Token C programming, ensuring security is paramount. Code vulnerabilities can lead to severe exploits, such as unauthorized access to user funds, exposure of sensitive data, or manipulation of the application’s logic. Given the decentralized nature of these systems, developers must consider a wide range of security factors when writing and deploying code to prevent malicious actors from exploiting any weakness in the system.
The design of token logic, cryptographic functions, and user authentication methods directly impacts the security posture of a distributed application. Even minor mistakes or oversights can create significant risks. The process of securing Token C code involves rigorous testing, code auditing, and ensuring that all interactions with the blockchain are well-guarded against common attacks, such as reentrancy or double-spending.
Common Security Risks in Token C Code
- Reentrancy Attacks: Occur when a contract calls an external contract before completing its current execution, allowing an attacker to re-enter the contract during the execution process.
- Integer Overflow/Underflow: Happens when a variable exceeds its defined limit, causing unintended behavior, like incorrect token transfers or balance calculations.
- Front-running: Refers to the act of executing transactions in an order that takes advantage of the knowledge of pending transactions in a blockchain network.
Best Practices for Secure Token C Code
- Use Safe Math Libraries: Leverage libraries that prevent integer overflows and underflows by implementing checks before performing arithmetic operations.
- Implement Proper Access Controls: Ensure that sensitive functions, like transferring tokens, can only be executed by authorized parties or contracts.
- Optimize Gas Consumption: Minimize the complexity of token transactions to avoid unexpected behavior from gas price fluctuations.
- Regular Audits: Conduct periodic code audits and security reviews to identify potential vulnerabilities before they are exploited.
Note: Token C code must be designed with the principle of least privilege in mind, limiting the permissions of users and smart contracts to only what is necessary for their operation.
Security Features in Token C Code Design
Security Feature | Purpose |
---|---|
Multisig Wallets | Require multiple signatures for performing sensitive actions, adding an extra layer of security. |
Time Locks | Prevent actions from being executed before a specified period, reducing the window for attacks. |
Encryption of Data | Ensure all sensitive data is encrypted both in transit and at rest to prevent unauthorized access. |